Hi there! If you’re looking to automate the process of adding devices to Netbox, using Python and the Netbox API is a great way to do it. In this post, I’ll walk you through the steps of adding a single device to Netbox using the pynetbox module.
To start, you’ll need to install the pynetbox module using pip.
pip install pynetbox
Once installed, you can begin using its methods to interact with the Netbox API.
The first step is to create an API object. This will act as the point of entry to the Pynetbox module and allow you to interact with the Netbox API.
nb = pynetbox.api(url='http://192.168.0.160/',
token='88a5fdbd9305b1c27b1bd6080b6d8c8da209e9b3')
Here, you’ll need to specify the base URL of your Netbox instance and your Netbox token. You can create a token using the Netbox admin panel.
Parameters:
• url (str) – The base URL to the instance of NetBox you wish to connect to.
• token (str) – Your NetBox token.
To create a token, use the Netbox administration panel.
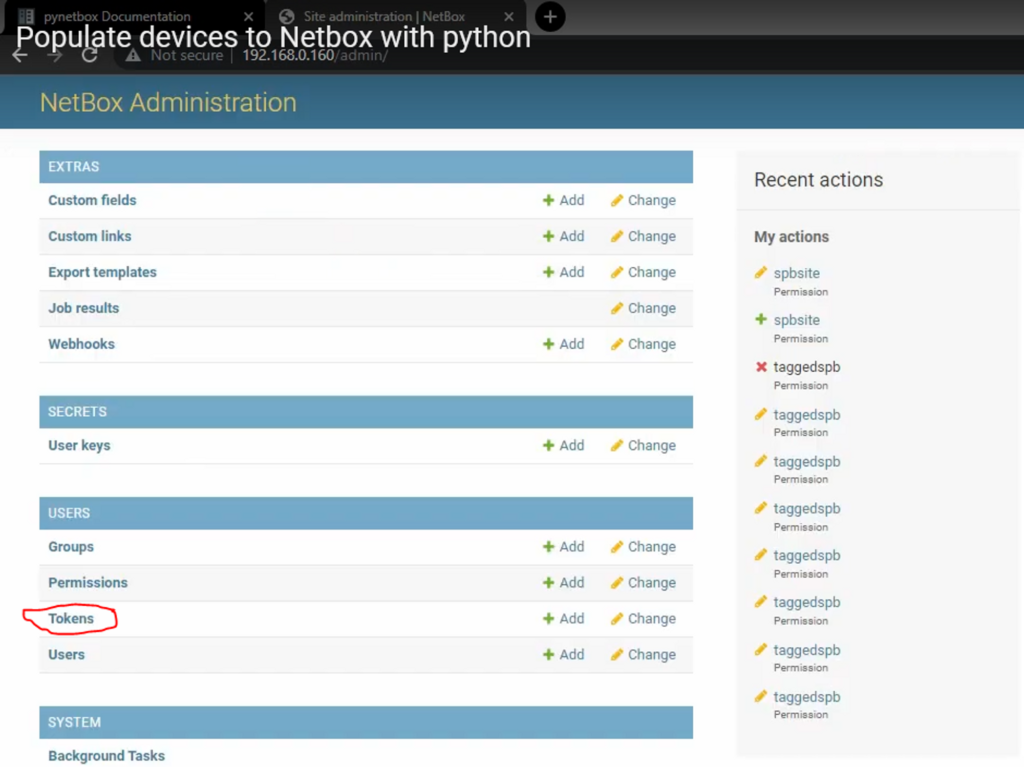
Next, click “Add Tokens”.

After creating the API object, we can use it to interact with the Netbox API and perform various operations. The “create” method is one such operation that can be used to add new objects to Netbox, such as devices, IP addresses, and circuits.
In our case, we used the “create” method to add a new device to Netbox. The required parameters, such as the device name, device type, device role, and site, were passed as named arguments. Once the method was executed, the new device was successfully added to Netbox.
Therefore, the “create” method is a powerful tool that can streamline the process of adding new objects to Netbox. By using the “create” method in conjunction with other methods available in the pynetbox module, such as the “update” and “delete” methods, we can efficiently manage our Netbox inventory.
In summary, we have created the “nb” object, which allows us to interact with the Netbox API, and have demonstrated the use of the “create” method to add a new device to Netbox. This method, along with others available in the pynetbox module, can greatly simplify the process of managing our Netbox inventory.
result = nb.dcim.devices.create(
name=dev,
device_type=6,
device_role=8,
site=7,
)
Here, we’re passing in the necessary parameters to create a new device. We’re specifying the device name, device type, device role, and site.
You can find detailed information on how to pass in these parameters and create a new device on netbox.dev. This documentation provides a comprehensive guide on how to use the Netbox API and Python to manage and automate the process of adding devices to Netbox.
To add a device, you must make a POST request.
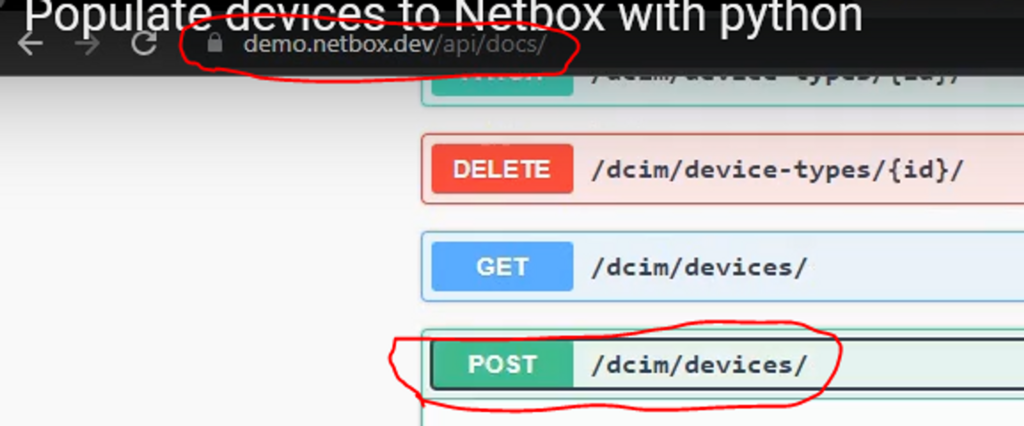
Find the “responses” section:
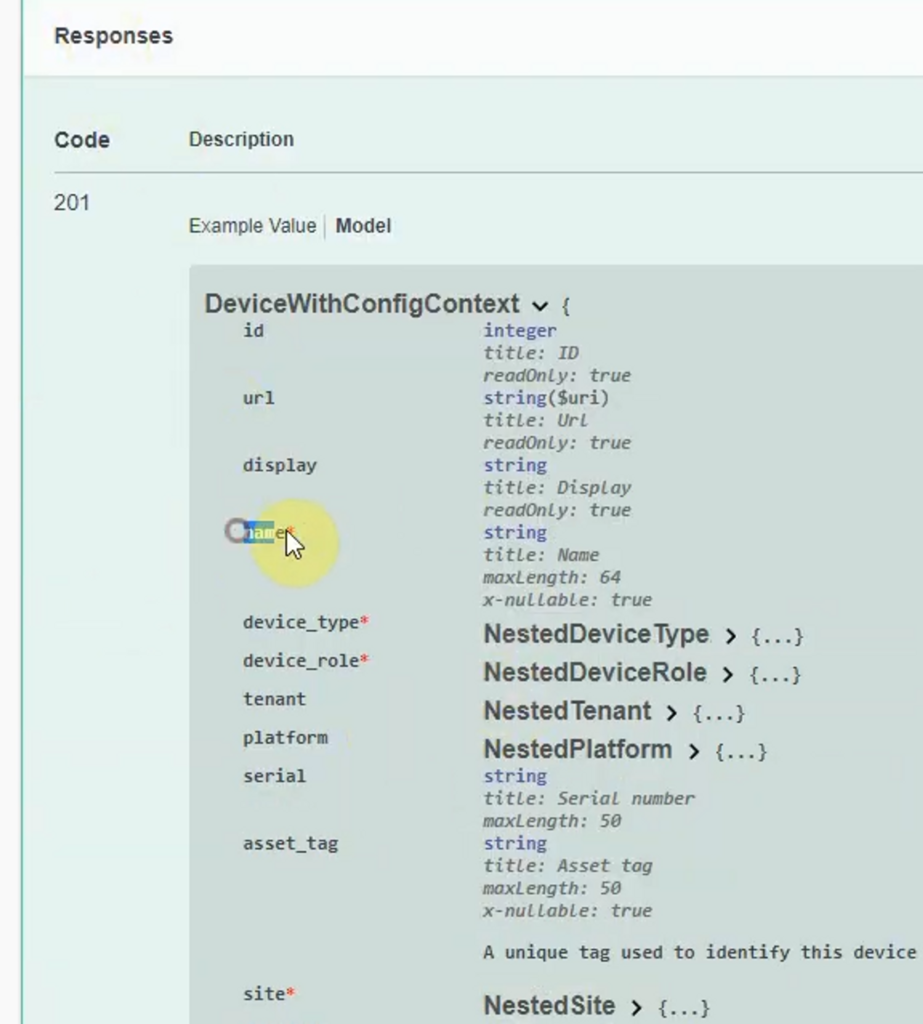
Necessary parameters are defined with a red asterisk.
Where did I get the necessary parameters: device type = 6, device role = 8, site = 7.
Go to Netbox UI:
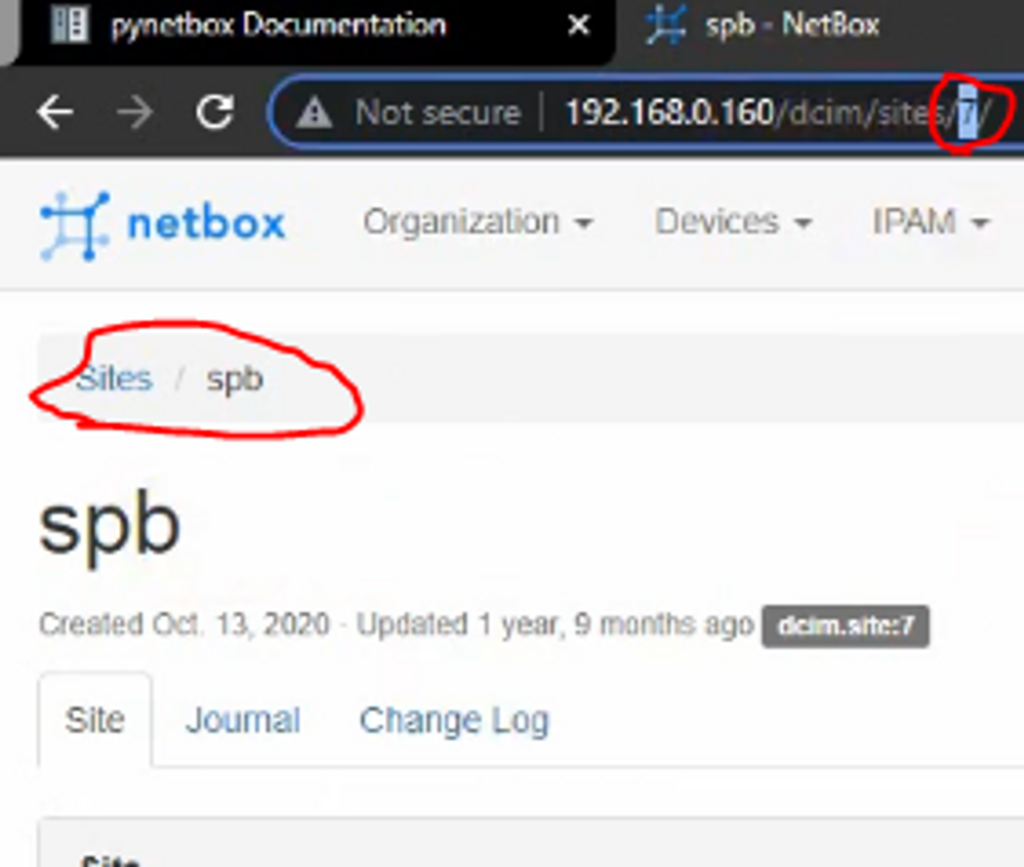
My site name is spb and site id is 7.
You need to perform the same actions for ‘device_type’ and ‘device_role’ as well.
And that’s it! Once you run this code, you should see the new device added to your Netbox instance. If you have multiple devices to add, you can use a loop to automate the process.
import pynetbox
dev = "R1"
def populating_devices(dev):
nb = pynetbox.api(url='http://192.168.0.160/',
token='88a5fdbd9305b1c27b1bd6080b6d8c8da209e9b3')
result = nb.dcim.devices.create(
name=dev,
device_type=6,
device_role=8,
site=7,
)
print(result)
populating_devices(dev)
I hope this post was helpful in getting you started with adding devices to Netbox using Python and the Netbox API. If you have any questions, feel free to leave them in the comments below.